Application note
Digital Output, application examples
Automatic door control:
Featuring: Maximum current monitor.
A gear motor is used to open and close a door. The motor is connected to an output. Direction is selected by a second output. Each time the motor is switched on; it draws 1.5A for a maximum of 0.5 second to initiate the movement. When the door is moving, it draws a maximum of 0.6 A. If the door hits an obstacle or has reached its end position, the current increases above 0.6A.
If the current is below 0.2A an alarm is generated: “motor out of order!”
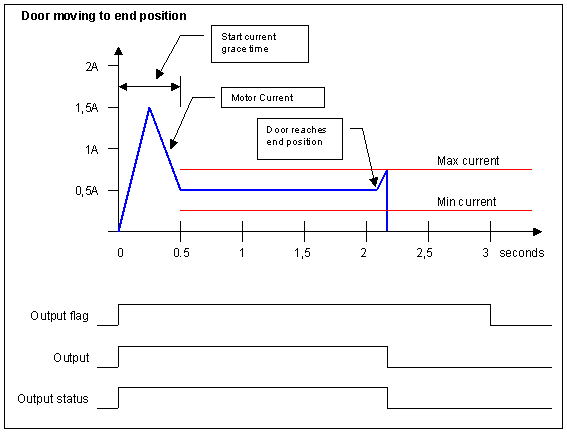
Software needed:
IF OpenDoorKey = Pressed Then
Begin
DoorDirection:= Open;
DoorMotor:= Start;
While OutputStatus = True Do Nothing; (* wait for max current *)
DoorMotor:= Stop;
End;
Detecting a blown bulb or Detecting a wire broken
Featuring: Minimum current monitor.
Connected devices normally have a minimum current when activated.
Automatically monitoring the current in the output can generate an alarm if e.g. a bulb is blown or a wire is broken to a device.
In this example, the output channel is configured to monitor for a minimum current of 200mA at 0.5 seconds after activating the output.
If the current is below 0.2A, an alarm is generated: “Temperature alarm light bulb blown!”
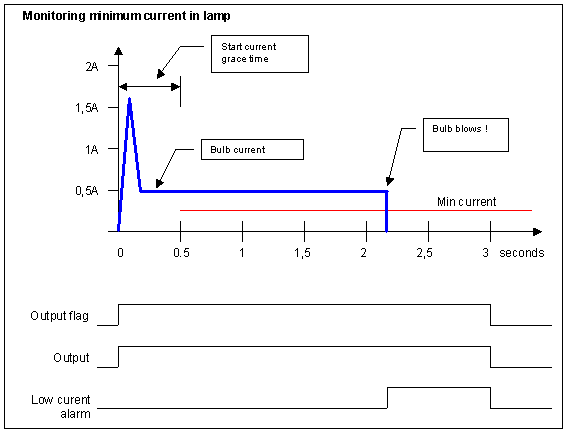
Software needed:
IF Temperature > MaxTemperature Then
Begin
AlarmBulb := ON;
IF AlarmBulb.LowCurrentAlarm = ON Then
SendAlarm(“High temperature alarm bulb is blown !”);
End
Else
AlarmBulb := OFF;
Dosing by fixed time base
Featuring: One shot output with fixed On time.
Dosing of a recipe component with a dosing pump into a mixing tank on a weight cell.
The dosing pump is controlled ON / OFF and the dosed amount depends on the ON time of the dosing pump. The output channel is configured as a one shot with a time to hold the output ON. When this time expires, the output switches to an OFF state. Timing is performed locally at the output channel and is thus completely independent of the system’s response time.
The Output status flag shows when a dose has been completed. If further dosing is needed, the output is re-activated, which will then generate another ON pulse.
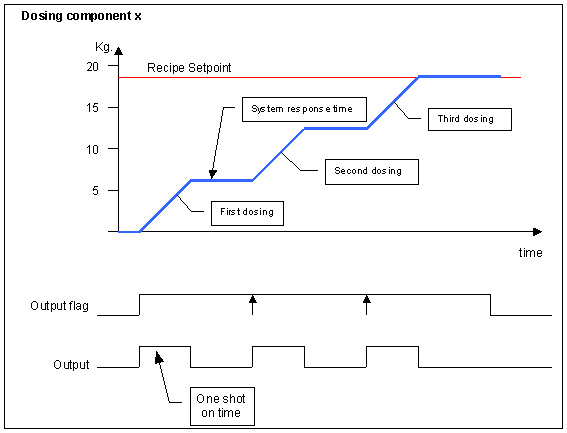
Software needed:
Repeat
OutputControlFlag := ON; (*initiate one pulse*)
While (OutputStatusFlag = True) Do Nothing; (*wait for finished *)
Until Weight >= RecipeSetpoint; (*finished ? *)
OutputControlFlag := OFF; (*reset the output *)
Dosing by adjustable time base
Featuring: One shot output with dynamically adjusted On time.
Dosing of recipe components with a dosing pump into a mixing tank on a weight cell.
The dosing pump is controlled ON / OFF and the dosed amount depends on the ON time of the dosing pump. The controller does a calculation of the dosing time, and configures the output channel to stay ON for the requested period. Timing is performed locally at the output channel, and is thus completely independent of the system’s response time.
The Output status flag shows that a dose has been completed. If further dosing is needed, a new calculated dosing time is written into the output channel’s one shot register, which then activates the output for the requested period.
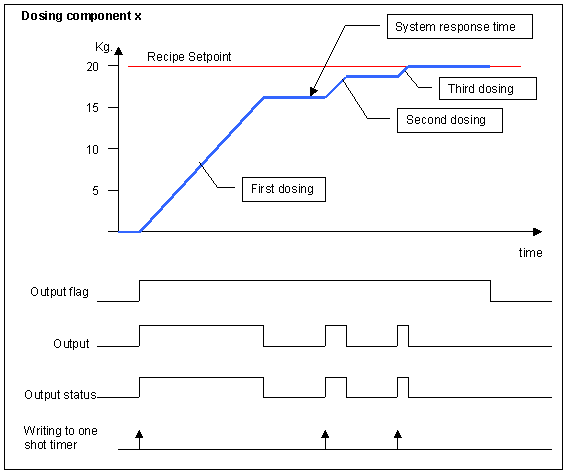
Software needed:
Repeat
OutputControlFlag := ON; (*initiate one pulse*)
While (OutputStatusFlag = True) Do Nothing; (*wait for finished *)
Until Weight >= RecipeSetpoint; (*finished ? *)
OutputControlFlag := OFF; (*reset the output *)
Dosing by pulses
Featuring: Duty cycle output, with dynamic control.
Dosing of recipe components with a stroke-dosing pump into a mixing tank on a weight cell.
The stroke dosing pump is controlled by an ON / OFF action. The dosed amount depends on the number of ON/OFF cycles.
The dosing frequency is high when the actual weight is significantly less than the set point. The frequency decreases as the gap between weight and set point narrows.
The output ON time is set to the minimum time for the stroke pump to operate. By changing the Total time, the frequency of the strokes can be adjusted. The minimum Total time is 2 x the one shot ON time to allow the stroke pump to return (50% duty cycle).
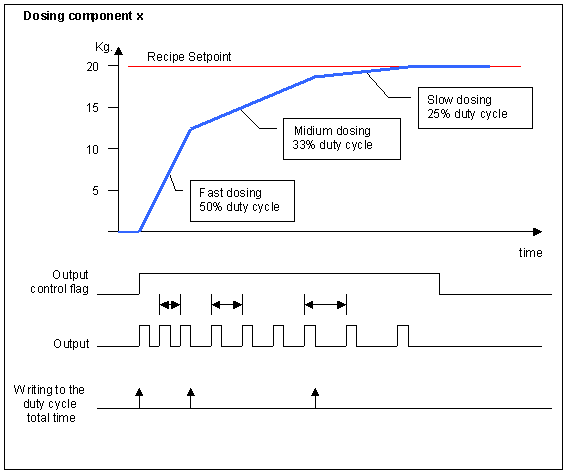
Software needed:
OutputControlFlag := ON;
While (Weight < Recipe Setpoint) Do
Begin
OutputTotalTime := CalculateDosingSpeed;
End;
OutputControlFlag := OFF;
Room light control
Featuring: Toggle mode and input hold time.
The light in a room is operated by a number of distributed push buttons to switch the light ON or OFF.
When any of the push buttons is activated, the light must be switched to the opposite of the current state.
At a fixed time of the day, all lights must be switched off.
The output channel is configured as Toggle Mode.
The input channel from the push buttons is configured as Input hold mode, which ensures the input is present for a period of time, allowing the controller to scan it and to avoid the light being switched on/off repeatedly if a key is pressed rapidly. (Alternatively the input could be handled by the notification system)
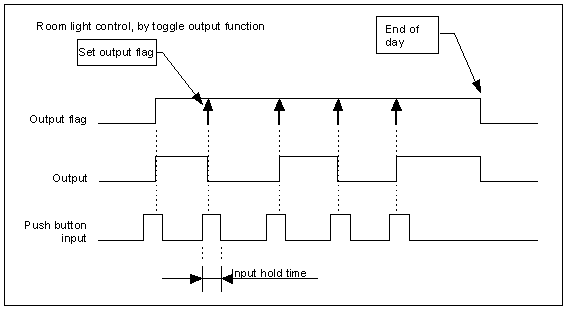
Software needed:
If PushButton = Pressed Then
Begin
OutputFlag := ON; (*toggle light*)
While (PushButton= Pressed) DO Nothing; (*wait Button released*)
End;
IF Time > EndOfDay Then
OutputFlag := OFF;
Room temperature control
Featuring: Duty cycle output, with dynamic control.
If a manually adjustable radiator thermostat controls the room temperature, an automation add-on can be created.
A resistor is placed under the thermostat. If power is dissipated in the resistor, it heats up and the thermostat will “believe” the room temperature is rising and thus lower the heat from the radiator.
The heat in the resistor is controlled by duty cycle output control. The higher the duty cycle, the hotter the resistor gets, and the more the thermostat lowers the room temperature.
In the example shown, the ON time is constant, and only the total time is adjusted. Of coarse, both parameters can be adjusted if needed, e.g. to get a 100% duty cycle.
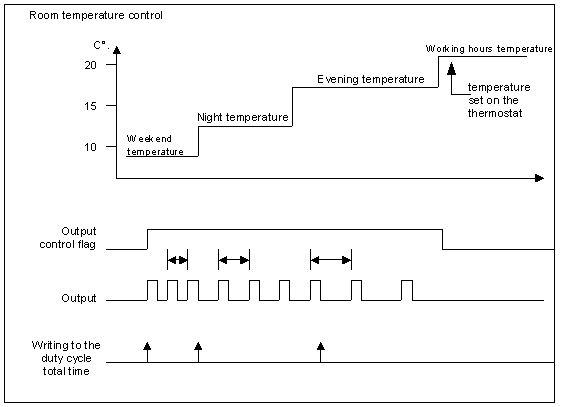
Software needed:
If WorkingHours then
OutputControlFlag := OFF (* enable normal thermostat control*)
Else
Begin
OutputControlFlag := ON
Case CurrentTime OF
Evening: OutputTotalTime := EveningTiming;
Night : OutputTotalTime := NightTiming;
WeekEnd : OutputTotalTime := WeekEndTiming;
End;
End;